Building Real-Time Applications with WebSocket in NestJS
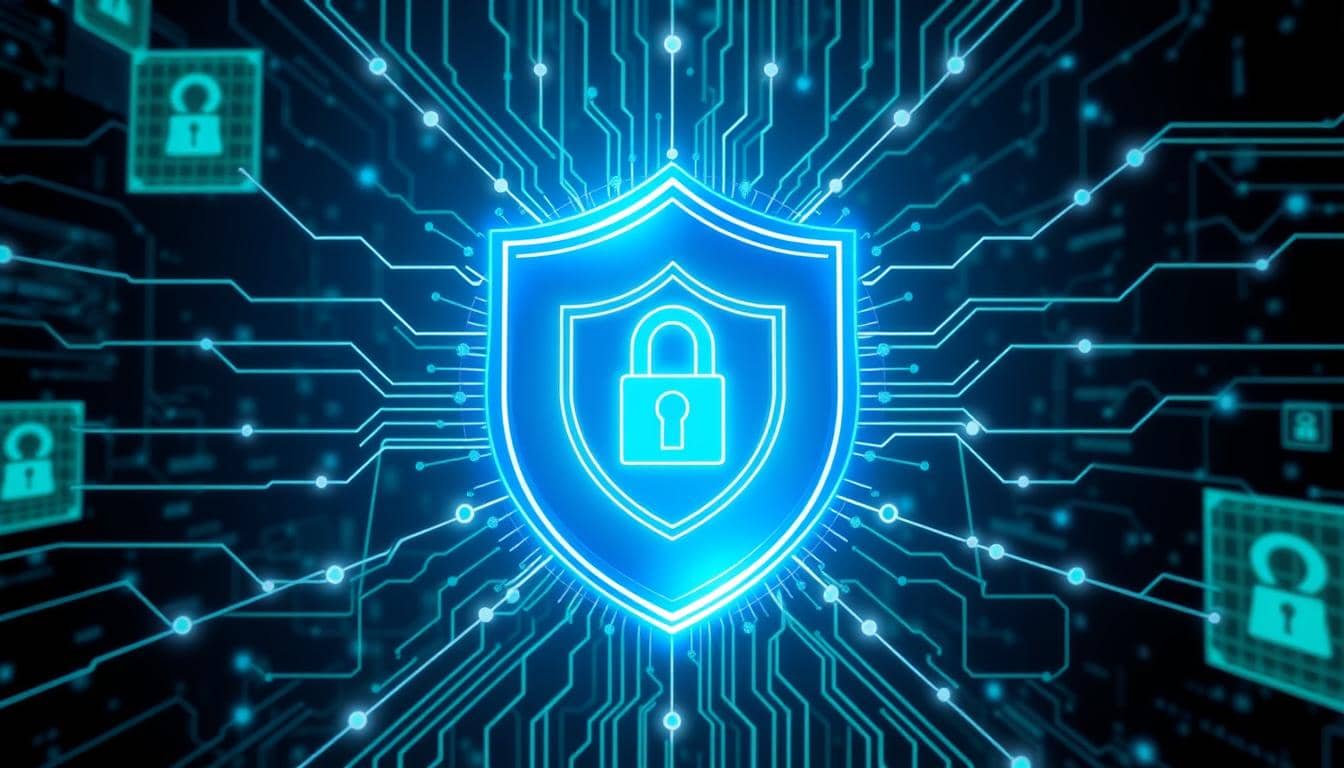
Contents
- 1 Introduction to Real-Time Applications
- 2 WebSocket Technology
- 3 Building Real-Time Applications
- 4 Handling Real-Time Events
- 5 Scaling Real-Time Applications
- 6 Security Considerations
- 7 Best Practices and Performance Optimization
- 8 Integrating with Third-Party Services
- 9 Use Cases and Examples
- 10 FAQ
- 10.1 What are real-time applications?
- 10.2 What are the benefits of real-time applications?
- 10.3 What is WebSocket technology?
- 10.4 How do I set up a NestJS environment for building real-time applications?
- 10.5 How do I handle real-time events in my NestJS application?
- 10.6 How can I scale my real-time application built with NestJS?
- 10.7 What security considerations should I keep in mind for my real-time application?
- 10.8 What are some best practices and performance optimization techniques for real-time applications built with NestJS?
- 10.9 How can I integrate my NestJS-powered real-time application with third-party services?
- 10.10 Can you provide some use cases and examples of real-time applications built with NestJS?
- 11 Author
Building Real-Time Applications ,In today’s fast-paced digital landscape, the demand for responsive and interactive web applications has grown exponentially. Real-time applications, capable of delivering instant data updates and seamless user experiences, have become the hallmark of modern web development. This article will explore how to leverage the power of WebSocket technology, combined with the robust NestJS framework, to create cutting-edge real-time applications that captivate users and drive business success.
Key Takeaways
- Understand the fundamentals of real-time applications and their benefits
- Explore the WebSocket protocol and its advantages over traditional HTTP
- Learn how to set up a NestJS environment and integrate WebSocket functionality
- Discover techniques for handling real-time events and scaling your real-time applications
- Gain insights into security considerations and best practices for optimizing performance
Introduction to Real-Time Applications
In the digital era, real-time applications have become increasingly prevalent, transforming the way we interact with technology. These applications are designed to respond to user input or external events instantly, providing a seamless and dynamic user experience. Understanding the nature of real-time applications and their numerous benefits is crucial for both developers and users alike.
What are Real-Time Applications?
Real-time applications are software programs that can process and act on information as it is received, without any noticeable delay. Unlike traditional applications that may require users to refresh or manually update content, real-time applications continuously monitor and update their interfaces, ensuring that the information displayed is always current and accurate.
Benefits of Real-Time Applications
The advantages of real-time applications are numerous and far-reaching. These applications offer:
- Improved User Engagement: By providing immediate feedback and updates, real-time applications keep users engaged and invested in the application’s functionality.
- Faster Information Exchange: Real-time applications enable the rapid exchange of information, allowing users to stay up-to-date on the latest developments and make informed decisions in a timely manner.
- Enhanced Collaboration: Real-time applications facilitate seamless collaboration by enabling multiple users to interact with the same data simultaneously, fostering better communication and decision-making.
Whether it’s a chat application, a stock trading platform, or a real-time monitoring system, the benefits of real-time applications are clear. By leveraging the power of technology, these applications have the potential to revolutionize the way we work, communicate, and interact with the digital world.
WebSocket Technology
In the dynamic world of modern web applications, the need for real-time communication has become increasingly crucial. WebSocket technology emerged as a game-changer, offering a seamless way to build applications that require instant data exchange between clients and servers. Unlike traditional HTTP protocols, which rely on a request-response model, WebSocket provides a bidirectional communication channel, enabling real-time, efficient, and low-latency data transfer.
At its core, WebSocket is a computer communications protocol that establishes a persistent, two-way connection between a client and a server. This persistent connection allows for continuous, real-time data exchange, making it an ideal choice for building applications that require instant updates, such as chat applications, real-time notifications, and online collaboration tools.
Feature | Benefit |
---|---|
Bidirectional Communication | Enables real-time, two-way data exchange between the client and server |
Low Latency | Minimizes the delay between the client’s action and the server’s response |
Persistent Connection | Maintains a continuous, long-lived connection, reducing the need for repeated handshakes |
Reduced Overhead | Requires less overhead compared to traditional HTTP-based communication |
By leveraging the power of WebSocket technology, developers can create real-time applications that provide a seamless and engaging user experience, transforming the way users interact with web-based services.
“WebSocket is a computer communications protocol, providing a way for a server and client to open a two-way interactive communication session over a single TCP connection.”
Building Real-Time Applications
Crafting real-time applications requires a robust development environment that can handle the complexities of real-time communication. In this section, we’ll explore the process of setting up a NestJS environment and delve into the implementation of WebSocket functionality to enable seamless real-time interactions.
Setting Up NestJS Environment
NestJS, a progressive Node.js framework, provides an excellent foundation for building real-time applications. To get started, you’ll need to have Node.js and npm (Node Package Manager) installed on your system. Once you have these prerequisites in place, follow these steps to set up your NestJS environment:
- Install the NestJS CLI globally using the following command:
npm install -g @nestjs/cli
- Create a new NestJS project by running the command:
nest new my-real-time-app
- Navigate to the project directory:
cd my-real-time-app
- Start the development server:
npm run start
Implementing WebSocket in NestJS
With the NestJS environment set up, it’s time to integrate WebSocket functionality into your real-time application. NestJS provides a dedicated WebSocket module, making the integration process seamless. To implement WebSocket in your NestJS application, follow these steps:
- Install the required dependencies:
npm install --save @nestjs/websocket @nestjs/platform-socket.io socket.io
- Import the WebSocket module in your application’s main module:
@Module({ imports: [WebSocketModule] })
- Create a WebSocket gateway to handle real-time communication:
@WebSocketGateway() class ChatGateway {}
- Implement the necessary event handlers to emit and listen to real-time events.
By setting up the NestJS environment and integrating WebSocket functionality, you’ll be well on your way to building robust, real-time applications that can handle dynamic and interactive user experiences.
Handling Real-Time Events
In the dynamic world of web applications, the ability to handle real-time events is a game-changer. With NestJS, you can seamlessly integrate WebSocket technology to create highly interactive and responsive applications. This section will explore the mechanisms for emitting events from the server and listening for those events on the client-side, empowering you to manage and respond to real-time data updates effortlessly.
Emitting Events
Emitting events is the cornerstone of real-time communication in your NestJS application. By leveraging the WebSocket protocol, you can push data updates from the server to the connected clients in real-time. NestJS provides a robust set of tools to make this process straightforward and efficient. You can emit events using the @SubscribeMessage()
decorator and the socket.emit()
method, allowing you to transmit data updates as they occur.
Listening to Events
On the client-side, it’s essential to set up event listeners to capture and respond to the real-time data updates from the server. NestJS simplifies this process by providing the @SubscribeMessage()
decorator, which allows you to define event handlers that are automatically invoked when the corresponding events are received. By listening to these events, your application can update its UI, trigger specific actions, and maintain synchronization with the server.
Together, the abilities to emit events and listen to events form the backbone of handling real-time events in your NestJS application. By mastering these techniques, you can create truly interactive and responsive experiences for your users, delivering a seamless and engaging real-time application.
“The true sign of intelligence is not knowledge but imagination.” – Albert Einstein
Scaling Real-Time Applications
As your real-time application gains traction and your user base expands, ensuring its scalability becomes paramount. Scaling real-time applications requires a strategic approach to handle the increasing demands on your infrastructure. In this section, we’ll explore proven techniques to scale your WebSocket-based application, including load balancing, message queuing, and the utilization of microservices.
Load Balancing for High-Traffic Scenarios
One of the essential strategies for scaling real-time applications is implementing a robust load balancing solution. By distributing incoming WebSocket connections across multiple server instances, you can ensure that your application can handle spikes in user activity without compromising performance. Load balancers can utilize various algorithms, such as round-robin or least-connection, to allocate traffic evenly and efficiently.
Leveraging Message Queuing
As your real-time application grows, it’s crucial to manage the flow of real-time events and messages. Message queuing systems, such as RabbitMQ or Apache Kafka, can help you decouple the processing of these messages from the WebSocket connections. By offloading message processing to a dedicated queue, you can prevent bottlenecks and ensure that your application remains responsive, even under heavy load.
Microservices for Scalable Architecture
Adopting a microservices architecture can significantly enhance the scalability of your real-time application. By breaking down your application into smaller, independent services, you can scale each component individually, allowing you to allocate resources more efficiently and respond to changing demand patterns. This approach also promotes modularity and flexibility, making it easier to maintain and update your application over time.
Technique | Description | Benefits |
---|---|---|
Load Balancing | Distributing WebSocket connections across multiple server instances | Handles spikes in user activity, ensures high performance |
Message Queuing | Offloading real-time event processing to a dedicated queue | Prevents bottlenecks, maintains application responsiveness |
Microservices | Dividing the application into smaller, independent services | Enables individual scaling, promotes modularity and flexibility |
By implementing these strategies, you can effectively scale your real-time application to accommodate growing user demands and ensure a seamless, high-performance experience for your users.
“Scaling real-time applications is a critical challenge that requires a strategic and multi-faceted approach. By leveraging load balancing, message queuing, and microservices, you can build a robust and scalable WebSocket-based infrastructure that can adapt to the evolving needs of your users.”
Security Considerations
In the realm of real-time applications built with NestJS and WebSocket technology, security is a paramount concern. As these applications often handle sensitive data and real-time user interactions, it is crucial to implement robust authentication and authorization mechanisms to safeguard the integrity and privacy of your data exchanges.
Securing your real-time application begins with reliable authentication processes. This ensures that only authorized users can access and interact with your application. Some common authentication methods include:
- JSON Web Tokens (JWT) for token-based authentication
- OAuth 2.0 for delegated authorization
- Multi-factor authentication (MFA) for enhanced security
Complementing the authentication process, authorization controls determine the specific actions and resources that each authenticated user is permitted to access. This granular access management helps mitigate the risks of unauthorized actions and data breaches.
Authentication Method | Description | Advantages |
---|---|---|
JSON Web Tokens (JWT) | A standard for securely transmitting information between parties as a JSON object | Stateless, scalable, and easy to integrate |
OAuth 2.0 | An open standard for authorization, allowing users to grant limited access to their resources | Delegated authorization, reduced complexity, and improved user experience |
Multi-factor Authentication (MFA) | An authentication method that requires the user to provide two or more verification factors to gain access | Increased security, protection against password-based attacks, and compliance with industry standards |
By incorporating these security considerations into your NestJS-based real-time applications, you can ensure the security considerations and authentication and authorization processes are robust, protecting your users and their data from potential threats.
Best Practices and Performance Optimization
When it comes to building real-time applications with NestJS, following best practices and focusing on performance optimization are crucial for ensuring the success and longevity of your project. By implementing these strategies, you can create a reliable, scalable, and responsive application that delivers an exceptional user experience.
Message Compression
One of the key performance optimization techniques is message compression. Real-time applications often involve the exchange of large amounts of data, which can impact network bandwidth and increase latency. By applying compression algorithms, such as gzip or deflate, you can significantly reduce the size of the data being transmitted, leading to faster response times and improved overall performance.
Error Handling
Robust error handling is essential for the stability and resilience of your real-time application. Implement comprehensive error-handling mechanisms to gracefully handle and log any issues that may arise during WebSocket communication. This includes handling connection failures, message parsing errors, and other potential exceptions. By proactively addressing and mitigating these potential problems, you can ensure a smooth and reliable user experience.
Client-Side Caching
Leveraging client-side caching can greatly enhance the performance optimization of your real-time application. By caching frequently accessed data on the client-side, you can reduce the number of roundtrips to the server, leading to faster response times and a more responsive user interface. Carefully consider the data that can be cached, such as user preferences, historical chat messages, or real-time updates, and implement efficient caching strategies to optimize the application’s overall best practices.
“Optimizing performance is not just about making things faster; it’s about creating an exceptional user experience that keeps your customers engaged and coming back.”
By following these best practices and implementing performance optimization techniques, you can ensure that your NestJS-based real-time application is reliable, scalable, and responsive, providing users with a seamless and engaging experience.
Integrating with Third-Party Services
Real-time applications often need to seamlessly integrate with various third-party services, such as chat providers, notification systems, or real-time data sources. Integrating these external services can greatly enhance the functionality and user experience of your NestJS-powered real-time application.
One of the key advantages of integrating third-party services is the ability to leverage specialized features and expertise. For example, by integrating a popular chat API, you can quickly add robust messaging capabilities to your application without having to build the entire infrastructure from scratch. Similarly, integrating a notification service can simplify the process of delivering real-time alerts and updates to your users.
When it comes to integrating with third-party services, there are several important considerations to keep in mind. First and foremost, you’ll need to ensure that the integration is secure and reliable. This may involve implementing robust authentication and authorization mechanisms, as well as carefully managing the exchange of data between your application and the external service.
Additionally, you’ll need to consider the performance implications of the integration. Real-time applications often require low-latency communication, so it’s important to choose third-party services that can deliver fast and efficient data exchange. Proper error handling and fallback mechanisms are also crucial to ensure a seamless user experience in the event of any service disruptions.
By carefully planning and implementing your integrations with third-party services, you can unlock a wealth of new possibilities for your NestJS-powered real-time application. From enhanced chat functionality to real-time data visualization and alerting, the opportunities are vast and can help you deliver truly engaging and valuable experiences to your users.
Third-Party Service | Potential Use Cases | Key Integration Considerations |
---|---|---|
Chat API |
|
|
Notification Service |
|
|
Real-Time Data Source |
|
|
“By seamlessly integrating with third-party services, you can unlock a wealth of new possibilities for your real-time application, delivering truly engaging and valuable experiences to your users.”
Use Cases and Examples
To provide a better understanding of real-time applications in action, this section will present several use cases and examples. We will explore the implementation of chat applications and real-time notification systems using the WebSocket capabilities in NestJS, showcasing how these technologies can be leveraged to create engaging and responsive user experiences.
Chat Applications
One of the most common use cases for real-time applications is the implementation of chat applications. By harnessing the power of WebSockets, NestJS allows developers to build real-time chat platforms that enable instantaneous message exchange between users. This technology can be employed to power features such as one-on-one conversations, group chats, and even live customer support interactions, providing users with a seamless and responsive communication experience.
Real-Time Notifications
Another compelling use case for real-time applications developed with NestJS is the implementation of real-time notification systems. These systems can be leveraged to deliver timely updates, alerts, and information to users as soon as they occur, without the need for constant polling or page refreshes. Examples of such real-time notification systems include stock price updates, breaking news alerts, and collaborative task management tools, all of which can be built using the WebSocket capabilities provided by NestJS.
FAQ
What are real-time applications?
Real-time applications are software programs that can respond to user input or external events immediately, without any noticeable delay. These types of applications provide a more engaging and responsive user experience.
What are the benefits of real-time applications?
The benefits of real-time applications include improved user engagement, faster information exchange, and enhanced collaboration. Real-time applications enable users to interact with the software in a more immediate and seamless way, improving the overall user experience.
What is WebSocket technology?
WebSocket is a computer communications protocol that provides a way for a server and client to open a two-way interactive communication session over a single TCP connection. This technology enables real-time, bidirectional communication between the client and server, making it an ideal choice for building real-time applications.
How do I set up a NestJS environment for building real-time applications?
To set up a NestJS environment for building real-time applications, you will need to follow the necessary steps to configure WebSocket functionality in your NestJS application. This includes installing the required dependencies, setting up the WebSocket server, and integrating it with your application’s logic.
How do I handle real-time events in my NestJS application?
To handle real-time events in your NestJS application, you will need to learn how to emit events from the server and listen for those events on the client-side. This involves understanding the mechanisms for managing and responding to real-time data updates in your NestJS application.
How can I scale my real-time application built with NestJS?
As your real-time application grows in user base and complexity, it’s essential to consider scalability. Strategies for scaling your WebSocket-based application include load balancing, message queuing, and the use of microservices to handle increasing real-time traffic.
What security considerations should I keep in mind for my real-time application?
Security is a critical aspect of any real-time application, especially when dealing with sensitive data or user interactions. You should focus on implementing robust authentication and authorization mechanisms to ensure the integrity and privacy of your real-time data exchanges.
What are some best practices and performance optimization techniques for real-time applications built with NestJS?
To ensure the success and fatcai99 longevity of your real-time application, it’s important to follow best practices and optimize performance. Techniques may include message compression, error handling, and client-side caching to improve the reliability, scalability, and responsiveness of your NestJS-based real-time application.
How can I integrate my NestJS-powered real-time application with third-party services?
Real-time applications often need to integrate with various third-party services, such as chat providers, notification systems, or real-time data sources. You can explore how to seamlessly integrate your NestJS-powered real-time application with external services, ensuring smooth and efficient data exchange and functionality.
Can you provide some use cases and examples of real-time applications built with NestJS?
To provide a better understanding of real-time applications in action, we will present several use cases and examples. This will include the implementation of chat applications and real-time notification systems using the WebSocket capabilities in NestJS, showcasing how these technologies can be leveraged to create engaging and responsive user experiences.